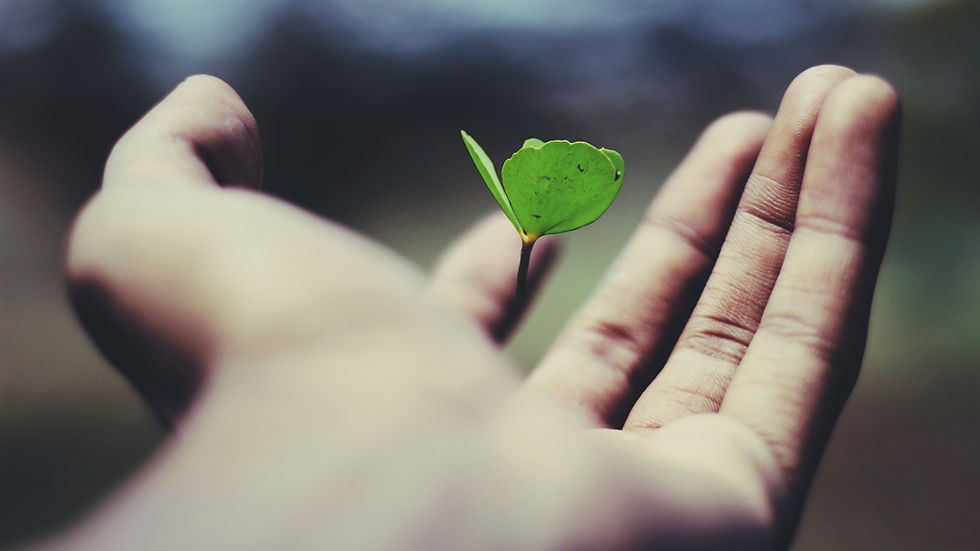
Concept
Lifecycle-aware components are useful for responding to changes in the lifecycle of activities and fragments. This organisation of code helps make it easier to maintain, because it is lighter-weight and well-structured. The usual pattern is to place the dependent components' actions within lifecycle methods. However, this can be inefficient and error-prone. By using these components, one can remove this code from the lifecycle methods into its own specific component. So, What we were doing till now?
The Activity has many responsibilities, as most of the code is placed within its lifecycle methods such as onCreate, onResume, and onPause. However, there are times when we need to take action depending on the activity's lifecycle.
Examples include accessing a user's location, playing videos, and downloading images.
The lifecycle of these actions is closely connected. If we need to access the user's location, On create must be used. Conversely, if the user presses the back button, then the location accessing process should be halted.
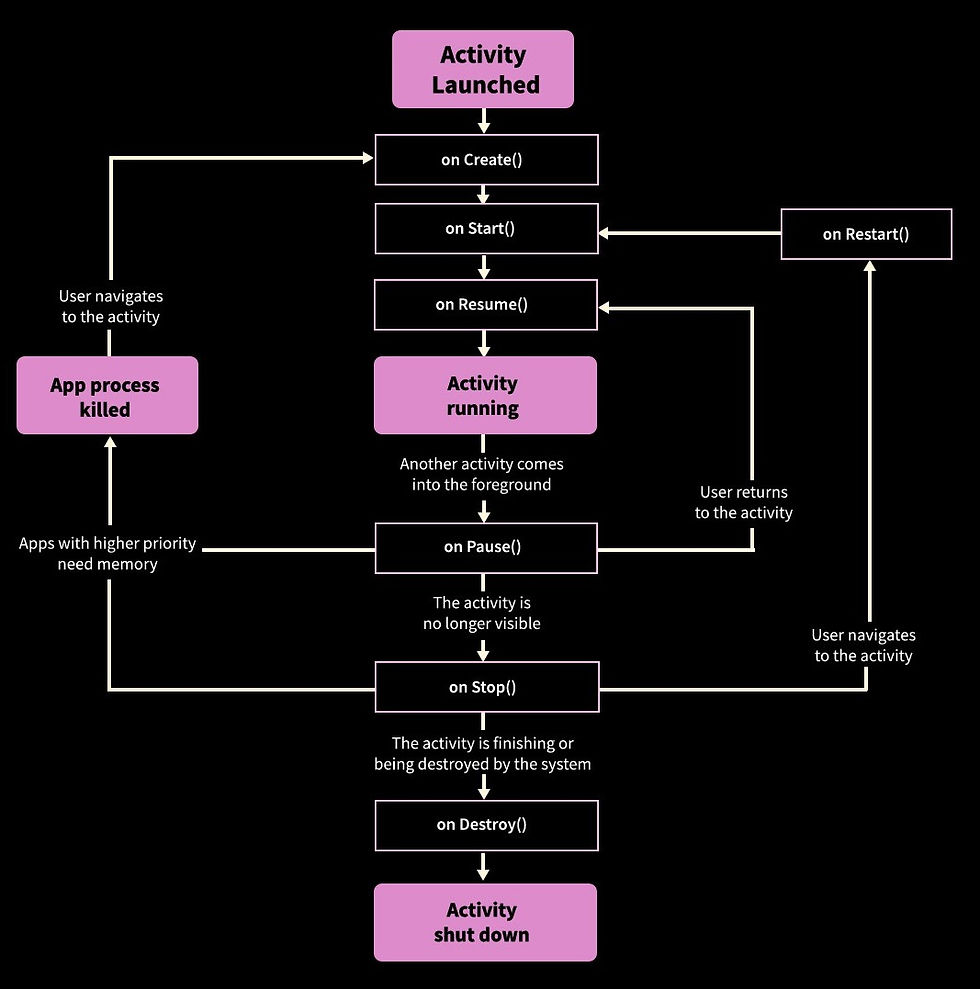
Package Responsible:
The androidx.lifecycle package offers classes and interfaces that facilitate dealing with issues in a stable and isolated manner. Lifecycle, which is a class that stores the information related to the lifecycle state of a component (e.g., activity or fragment), allows other objects to examine this state. To keep track of the life cycle status of its associated component, Lifecycle makes use of two primary enumerations:
Event, which maps to callback activities in activities and fragments and
State, which represents the current status of the component being tracked by Lifecycle object.
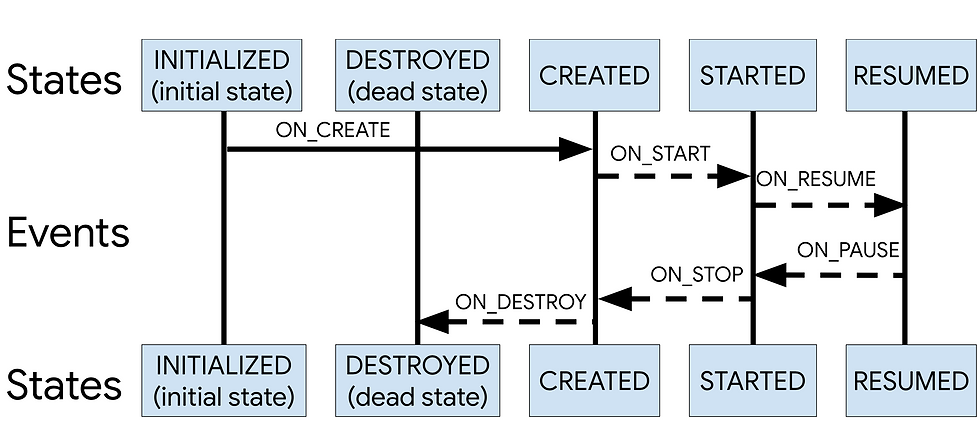
Put simply, Life Cycle components are elements that understand the stages of a android's life.
Breakdown of Life Cycle Components
Life Cycle Owner
Life Cycle Observer
As the name suggests, The life cycler owner is the one who owns the life cycle(e.g activities, fragments) and observer just sits and observe the life cycle.
Code Example
Open the Fresh Project in Android Studio with a JetCompose Activity and add life cycle dependencies.
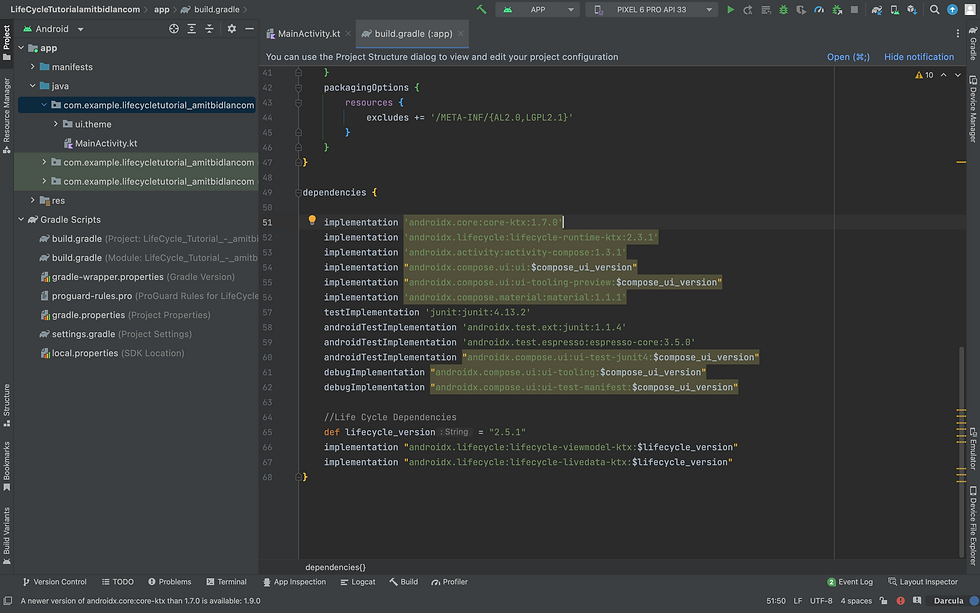
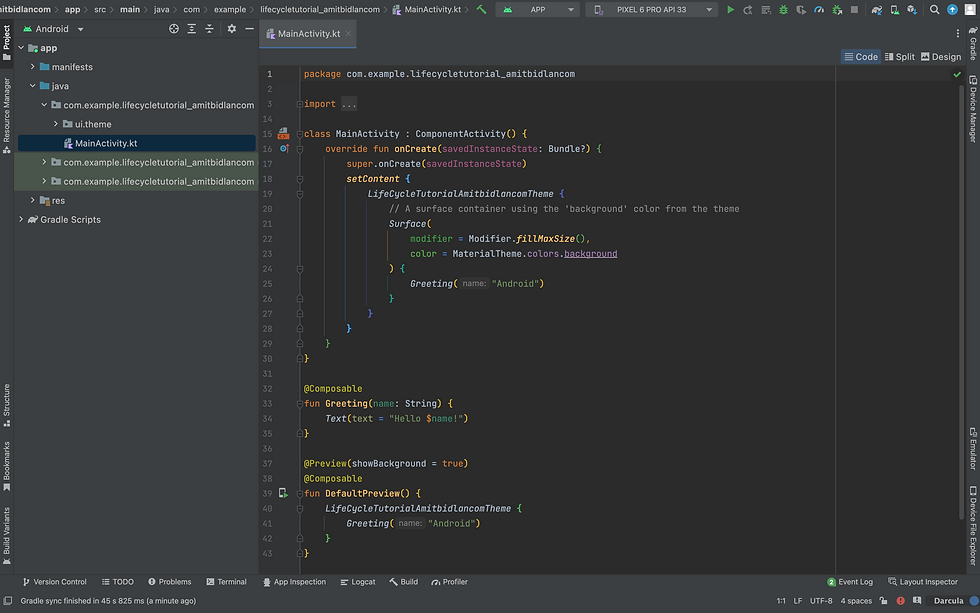
Now Let's Create a Kotlin Class named Observer for Our Ease.
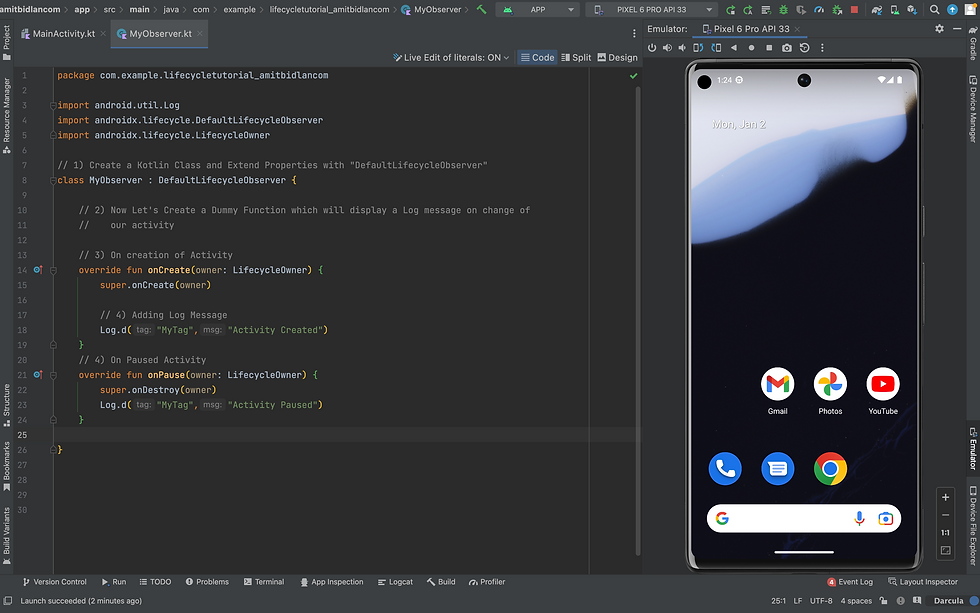
Now Let's add it to our Main Activity.

Results
On Activity Creation
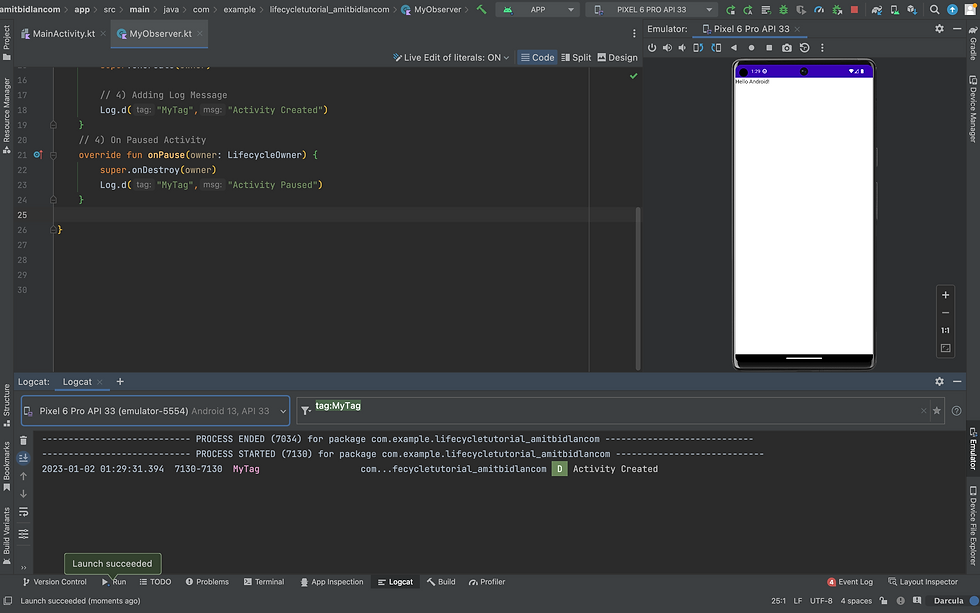
On Activity Pause

Copy Code From Here:
Complete Code
package com.example.lifecycletutorial_amitbidlancom
import android.util.Log
import androidx.lifecycle.DefaultLifecycleObserver
import androidx.lifecycle.LifecycleOwner
// 1) Create a Kotlin Class and Extend Properties with "DefaultLifecycleObserver"
class MyObserver : DefaultLifecycleObserver {
// 2) Now Let's Create a Dummy Function which will display a Log message on change of
// our activity
// 3) On creation of Activity
override fun onCreate(owner: LifecycleOwner) {
super.onCreate(owner)
// 4) Adding Log Message
Log.d("MyTag","Activity Created")
}
// 4) On Paused Activity
override fun onPause(owner: LifecycleOwner) {
super.onDestroy(owner)
Log.d("MyTag","Activity Paused")
}
}
// Inside Main Activity
lifecycle.addObserver(MyObserver())
Comments