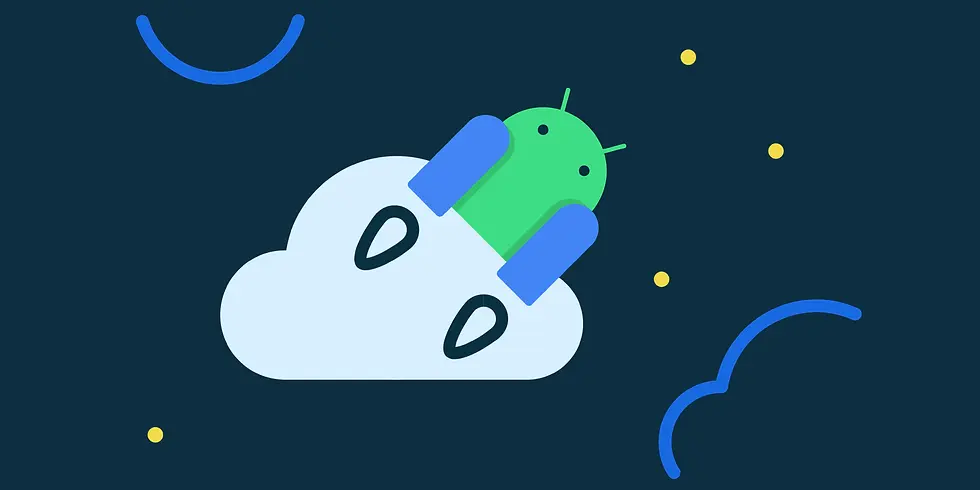
Understanding what a View Model is and how to use it in an Android app can be daunting. But with this post, we'll make the understanding simpler by using clear explanations and step-by-step instructions that will help you take advantage of this important component of app development.
What Are Android View Models?
View Model is an architecture component designed to store and manage data associated with the user interface. It helps separate business logic from the user interface, making it easier to test, debug, and upgrade apps at scale. View Models are primarily used for caching view-related data so that changes made to views can be immediately displayed when a user reaps the application.
When Should You Use a View Model?
View Models are great for UI-related data that needs to be preserved when the app is rotated, minimized, or paused. It is an ideal solution for tasks such as loading data from a remote server and displaying it on the screen. Additionally, a View Model can act as an event hub between views and their associated objects. This streamlines communication between the model and the view, ensuring consistent state throughout the application.
The Benefits of Using a View Model
One of the primary benefits of using a View Model is that data is retained throughout the application's lifecycle. This means that users don't lose their place if they switch to another app or rotate their device. In addition, View Models also provide a simplified way to communicate between model and view objects in an event-driven manner while allowing the UI thread to remain unblocked.
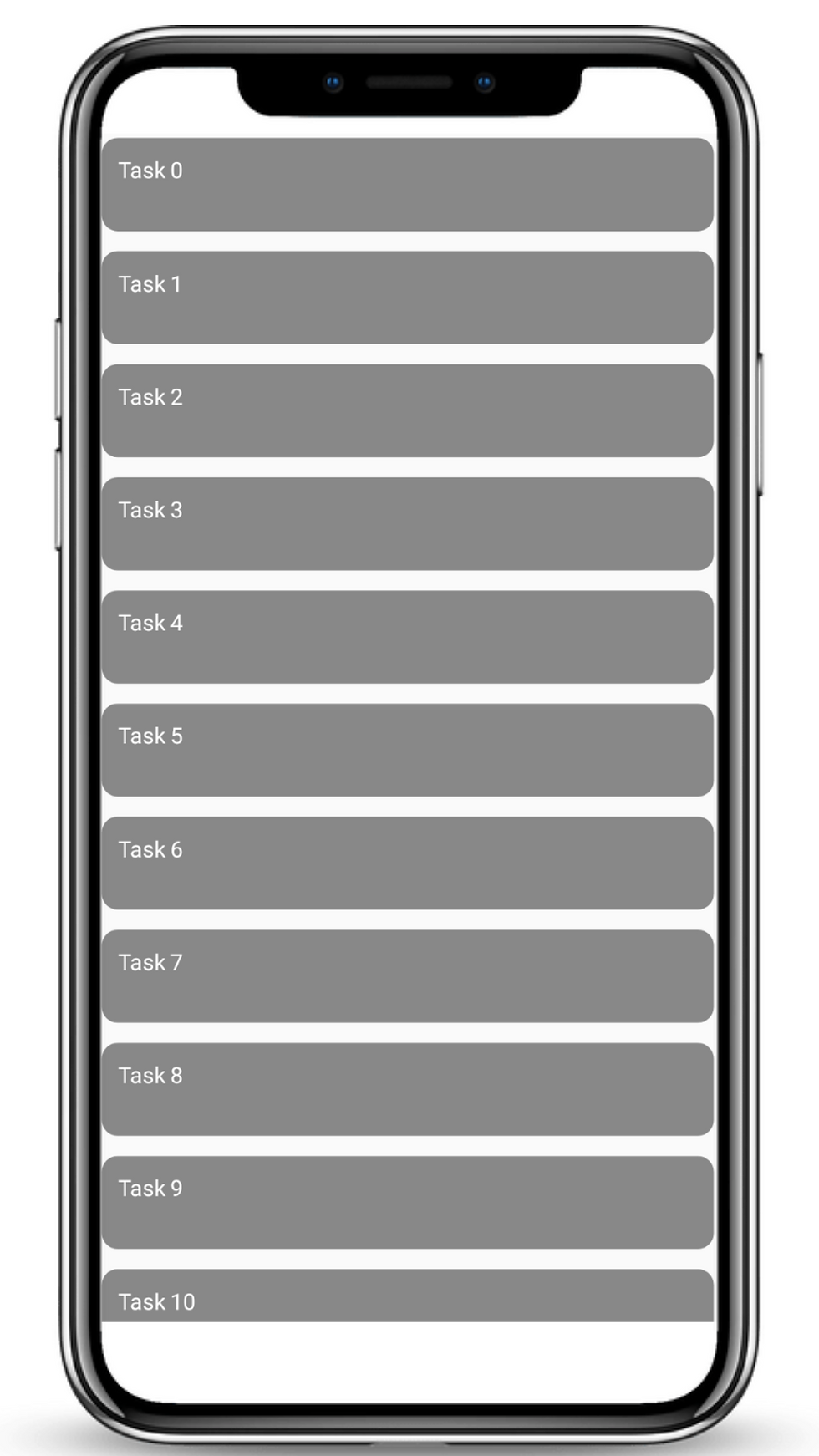
What We will Create?
We will be creating a list of task using Lazy Column and View Model.
Add Life Cycle and Live data dependencies.
Copy Dependencies from my Github repository.
Steps to Create a View Model in JetPack Compose 2023
Create a Data Class
Create a View Model Class
Link View Model data in Composable to Update on UI
Create a Data Class
data class DataClass(val id:Int, val label : String)
This Data class will be different according to your needs. Here my motive is to create a list of Data class which will provide me Task with Index.
Id - Will be taken as Index, and Label as Task Name.
Create a View Model Class
class DataClassViewModel : ViewModel() {
val _tasks = taskList().toMutableList()
}
private fun taskList() = List(30){
i -> DataClass(i,"Task $i")
}
This Data Class View Model extends the property of View Model class. First We create a List of data class of size 30. We will Convert this List to a mutable List.
Create a mutable list of data inside view model class.
Let's Link it from One Kotlin file to Another
Here comes the easy part. Relax, I got you. Unlike XML files we don't need to explicitly create a view model object and pass it main activity.
So Here I am creating a new Kotlin file named Home Screen where I am putting all UI elements.
@Composable
fun HomeScreen(modifier:Modifier = Modifier){
}
Let's pass the our View Model Class as an argument.
@Composable
fun HomeScreen(modifier:Modifier = Modifier,
dataClassViewModel: DataClassViewModel = viewModel()){
}
If your View Model Name class is something like, MyDataClass then the declaration suggestions as a function argument will be : myDataClass : MyDataClass = viewModel()
You may have noticed that the initial letter is lowercase, which is a standard in the Kotlin language.
Let's Create a Card to show our tasks. This is a preview of one item of our Task List.
@Composable
fun HomeScreen(modifier:Modifier = Modifier,
dataClassViewModel: DataClassViewModel = viewModel()){
}
@Composable
fun listcard(label: String, modifier: Modifier = Modifier) {
Card(
Modifier
.height(70.dp)
.fillMaxWidth()
.padding(6.dp)
.clip(shape = RoundedCornerShape(10.dp)), backgroundColor = Color.Gray) {
Text(text = label, modifier = Modifier.padding(10.dp), color = Color.White)
}
}
Play Around with this Listcard for more designing. Let's Create Lazy Column.
@Composable
fun HomeScreen(modifier: Modifier = Modifier,
dataClassViewModel: DataClassViewModel = viewModel()) {
LazyColumn(){
items(items = dataClassViewModel._tasks){
Task ->
listcard(label = Task.label)
}
}
}
@Composable
fun listcard(label: String, modifier: Modifier = Modifier) {
Card(
Modifier
.height(70.dp)
.fillMaxWidth()
.padding(6.dp)
.clip(shape = RoundedCornerShape(10.dp)), backgroundColor = Color.Gray) {
Text(text = label, modifier = Modifier.padding(10.dp), color = Color.White)
}
}
How We are accessing data now? Through our view Model. Refer to the data class.
We accessed the data by viewModel class.
If you are having problem with LazyColumn / Lazy Row . You can follow this You Tube Video.
All you need to replace LazyRow with LazyColumn.
Comments